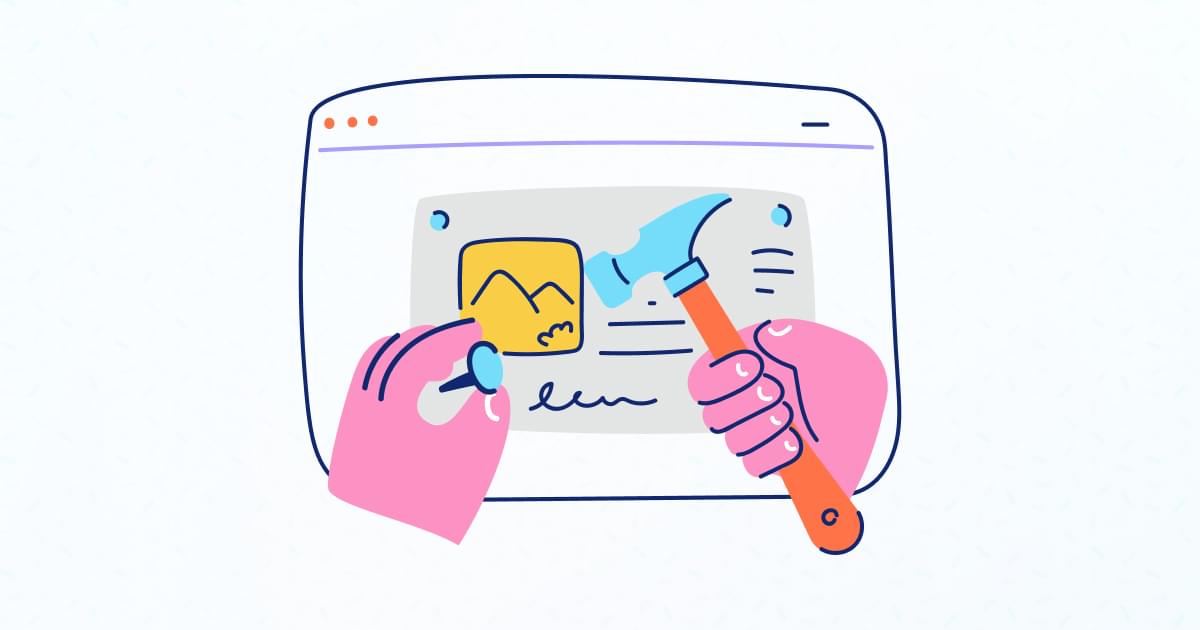
When it comes to app development, Apple offers developers one of the most cohesive experiences out there. With an intuitive and powerful coding language like Swift, developing for macOS and iOS is a breeze. But even if you’re new to coding, getting started with app development on a Mac or iPad is easy – especially with the wealth of resources available from Apple.
If you’re interested in developing your own Apple apps, this article is for you. We’ll walk you through the basics of development in the Apple ecosystem, and how to get set up with tools like Xcode and Swift. We’ll also show you how to test and debug your app before publishing it in the App Store.
By the end of this article, you’ll have everything you need to get started developing your own Apple ecosystem apps!
Set a High-Level Plan & Get Prepared
Table of Contents
The first step to learning a new skill set is always to form a plan, set some goals, and get the prerequisites prepared.
In the next steps, we’ll do some research and setup, and we’ll decide what we want to achieve as a measure of progress on the learning objectives we set next.
1. Set up your Apple developer account
If you plan to create an app, you need to create an Apple ID and join the Apple Developer Program.
2. Install Xcode and learn the basic interface
Xcode is a necessity in the Apple development ecosystem. Your goal by the end of this guide should be to have it installed and acquaint yourself with the basics of the interface enough to know roughly where to look for things.
3. Explore learning resources
Thanks to Swift’s open-source nature, you’ll always find a solution online whenever you get stuck. Swift’s source code, regular builds, mail lists, and bug tracker are publicly available. The learning resources help you understand how Swift works and provide valuable insights into other developers’ contributions.
Some of the resources include:
- Apple Swift Resource Guides
- Swift programming language e-Book
4. Successfully run a simple program
“Hello World!”
By now, you’re aware of this common greeting in the world of developers. But there’s more to this two-worded polite introduction.
While it’s a simple program that newbies can create, it establishes Swift syntax and structure knowledge. It also introduces you to Xcode, Apple’s programming environment.
We know you’re eager to develop a cutting-edge iOS app. But before then, a Hello World app should be your first brainchild.
5. Plan your first app
Planning the app that inspired you to start this journey in a little more detail will help you identify skills you’ll need to pick up along the way.
What do you want your app to do? For instance, do you want it to:
- Efficiently manage a task?
- Calculate something?
- Connect people?
- Make someone’s life easier or more fun in some way?
Planning helps you decide on the app’s features.
Search for inspiration online to see how other developers structure their Swift projects. GitHub can be your excellent place of inspiration and help. Here are a few Swift repos on GitHub worth checking out:
6. Understand the components of the Apple developer ecosystem
The Apple developer ecosystem is made up of many different components, including:
- The Xcode IDE
- The Swift programming language
- The SwiftUI user interface framework
- Developer tools like TestFlight, which allows developers to distribute test releases to a limited number of users
- Apple’s App Store facilities, such as App Store Connect
Each of these components plays a vital role in the development process, and you’ll need to have a good understanding of all of them in order to be successful.
More importantly, by getting familiar with the ecosystem from the top-down, you’ll have a better idea of where to look for help when you get stuck.
Now that we’ve set some goals, we’ll be able to get familiar with the Apple development world efficiently and maximize our learning.
Get to Know the Apple Developer Ecosystem
Familiarize yourself with the tools that will help you craft your first app. Of course, an apple-powered computer like MacBook or iPad should be your first tool of work. Let’s diver deeper:
The Xcode IDE
The Xcode IDE (Integrated Development Environment) brings all the tools you need under one roof. It’s where you write Swift code, create a user interface (UI), and link the data to your UI.
The Swift programming language
Swift is Apple’s programming language for on-platform development. The best part about Swift? It allows you to employ the same tools and frameworks to create apps for a variety of platforms, such as iOS, tvOS, iPadOS, macOS, and watchOS.
SwiftUI
SwiftUI is a user interface framework that makes coding UIs a breeze. It also helps you connect your UI to your app’s functional code. With SwiftUI, you can quickly and easily create complex interfaces without having to worry about the underlying code.
SwiftUI also includes a number of built-in components, such as buttons, labels, and text fields, that you can use in your app’s UI.
TestFlight
TestFlight is a valuable tool for developers that allows you to distribute test versions of your app to a limited number of users. This is a great way to get feedback on your app before releasing it to the general public.
AppStore Connect
With AppStore Connect, you can seamlessly publish and manage your apps on Appstore. A $100 annual fee is all you pay to access this portal and some other tools.
You feed the portal with your app’s details, such as name, screenshots, and description. It also allows you to upload the build bundle from XCode directly.
Done listing your app? Submit it from the portal directly and wait for review. Then cross your fingers as you wait for the app to go live on AppStore once it satisfies the reviewers.
Provisioning Portal
The signing tool is included in the provisioning portal, which allows you to sign your code. This way, Apple may identify you as the original author of your app. Users can also recognize your app as the original version. This keeps their security intact.
The Apple Developer Program allows you to distribute your brainchild worldwide. Do you want to develop an app just for yourself? In this case, you don’t have to join the program.
Diving Into Xcode IDE
Let’s familiarize ourselves with Xcode ID: Where to find it, kick-starting a new project, main Xcode interface parts, and your project file system. If you’re familiar with Apple software users, Xcode shouldn’t be strange to you.
Where to download Xcode
Finding and downloading Xcode is easy as pie. Just launch the Mac App Store, and look up Xcode. It should pop up as the first result. Lazy like me? Here’s a direct link to download and install Xcode without paying anything.
Did the app’s rating make you think twice? Just ignore them, as most of the reviews don’t make sense. Xcode is reputable software.
After downloading and opening the app, enter the admin password to download additional requirements.
Beginning a new project
After opening the app, select Create a new Xcode project.
You’ll then be presented will several templates. Make your choice and finish with a few other settings.
Let’s go with the app template for now and hit Next.
Provide your app’s information:
- Product name
- Team (for those with developer accounts)
- Organization identifier
Then choose the interface, lifecycle, and language. Select the SwiftUI interface and the SwiftUI App lifecycle.
Leave the checkboxes below (Use Core data and Include Tests) unchecked for now. Hit the Create the Project button and save it in an easy-to-recall place.
Exploring Your Project
Welcome to your new project. Don’t let the many panels and files intimidate you. In most cases, you’ll only need a few panels:
This panel has a listing of your project and the associated files on the far left. It also comes with various tabs shown by the small icons at the top. For starters, focus on the first tab.
Main/Editor area
Click a file in the navigator, and the editor area will display it, allowing you to edit the file.
The main area is adaptive to the file type, giving you the relevant editor you need. For instance, you’ll have the code editor if you choose a swift file. Asset picker would come up if you selected an assets file.
Inspector
Locate the Inspector area in the far right of the IDE. It displays either the configuration properties or the extra supplementary details (like documentation) of the component you select in the code editor.
Toolbar
The toolbar runs across the top of the window. It comes with several crucial functions.
The Play button helps you build and run your project. Want to halt the running project? Hit the square icon next to it.
You can select the simulator you desire using the picker over the editor section. And you can see the project’s compile status: Succeeded or failed.
The “+” button allows you to seamlessly add components to your app’s UI.
Your Project’s Files
An Xcode project’s file system looks as follows:
At the top, we have your project’s root folder. If you click on it, a metadata editor appears in the editor area, allowing you to edit your app’s metadata, such as name, bundle identifier, signing, and compatibility.
The “Preview Content” folder has all your project’s code files. Find your project’s output app (generated upon building and compiling your project) in the “product” folder. It stores your deployable bundle.
{NAME}App.swift
This file is your app’s starting point, named after your product. The “@main” tag below is your product’s entry point, where you begin processes.
It creates the ContentView() you see in the simulator. As seen, that calling function’s name maps to the “ContentView.swift” file.
ContentView.swift
This file contains your project’s UI code. You can open and edit it.
Assets.xcassets
This folder contains all your assets: Audio, images, video files, etc. So how do you add your desired assets to this folder? Just click the assets folder to reveal the asset manager and drag and drop the asset.
Building UI in SwiftUI
Building UI for your product is another hassle-free process. First, let’s learn how to preview the project to see how it appears when deployed.
Hit the Resume button to build your project and reveal its preview on an actual device.
Note the code portion from line 12 below. The preview shows the Text keyword with a “Hello World” in the braces. The modifier “.paddling()” (at the end) adds some spacing around the preview’s words.
So basically, all the UI elements can be in the () braces we highlighted above. Container elements come in here to make this possible.
Let’s recreate the starter code for Hello World from the ground up. Add the following code:
Upon updating the preview, here’s what we should have:
This simple Text element allows you to show text in the user interface.
You can add spacing around a user interface elements using the “.padding”() modifier. You can see a blue border around the text you wrote in the code.
Want to add some spacing to the text element? Insert the paddling modifier next to it.
Continue Learning to Code in the Apple Ecosystem
You’re now on the right footing to take the world of Apple apps by storm. But that’s just the tip of the iceberg. There’s plenty more to learn from here.
Sign up to SitePoint Premium free of charge and continue learning!