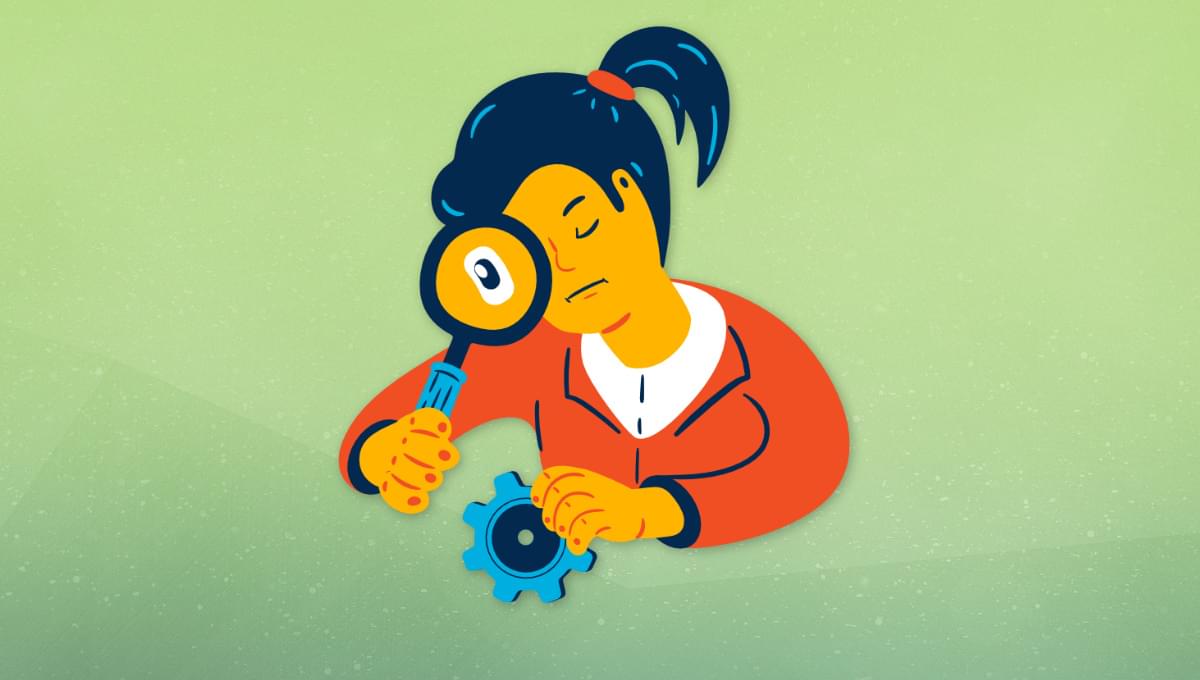
In this PHP quick tip, we’ll cover how to check whether or not variables are set, and if so, what their values are — which is a very common task in programming.
When checking if a variable is set in PHP, our first impulse might be to use the isset()
function. And while this will work for most cases, if we look at the definition of the isset()
function, we’ll see the following:
isset
: determines if a variable is declared and is other than null.
However, in PHP we can set a variable to be NULL
:
<?php $variable = NULL; var_dump(isset($variable));
This attribution will return false
— even though we’ve explicitly set our variable to NULL
! This is a condition within PHP that might generate some confusion. As we proceed below, we’ll look at the isset()
method and when to use it, and what other methods we can use when isset
doesn’t work.
Enter empty() and is_null()
While the isset()
method can help us determine if a variable has been set with some value, it won’t help us if the variable has been set with NULL
. To deal with this situation, we need to use either the empty()
or the is_null()
function. The empty()
function will determine whether a variable is empty. It will work (that is, return true
) for:
""
(an empty string)0
(0 as an integer)0.0
(0 as a float)"0"
(0 as a string)NULL
FALSE
array()
(an empty array)$var;
(a variable declared, but without a value)
This means we can use empty()
to determine if a variable is empty or not. And in this case, both NULL
and false
are considered empty:
<?php $variable = NULL; var_dump(empty($variable));
We can also use the is_null()
function. This function will determine whether a variable is null or not.
Here’s an example of using is_null()
to determine whether a variable is or isn’t null:
<?php $variable = NULL; var_dump(is_null($variable));
The most important thing when dealing with variables in PHP is to understand the differences between isset()
, is_null()
, and empty()
. These three functions can be used to determine the status of a variable, but they have different behaviors. The following table can be used to highlight the differences between the functions.
“” | “foo” | NULL | FALSE | 0 | undefined | |
---|---|---|---|---|---|---|
empty() | True | False | True | True | True | True |
is_null() | False | False | True | False | False | True (error) |
isset() | True | True | False | True | True | False |
Is There a Single Function We Can Use?
Using get_defined_vars()
will return an associative array with keys as variable names and values as the variable values. We still can’t use isset(get_defined_vars()['variable'])
here, because the key could exist and the value is still null, so we have to use array_key_exists('variable', get_defined_vars())
.
For example:
<?php $variable = null; $isset = array_key_exists('variable', get_defined_vars()); var_dump($isset); $isset = array_key_exists('otherVariable', get_defined_vars()); var_dump($isset);
By using get_defined_vars()
in this way, we can be 100% sure that we’re checking if the variable is set or not.
Conclusion
In this short article, we discussed how we can check whether a variable is set in PHP or not. We also looked at the differences between set, empty and null, and the key considerations to keep in mind when using the common functions isset()
, is_null()
, and empty()
. So, the next time you need to check the status of a PHP variable, you’ll have all the information you need so you can choose the right method and eliminate all ambiguities from your code.